Demand-driven APIs Using GraphQL
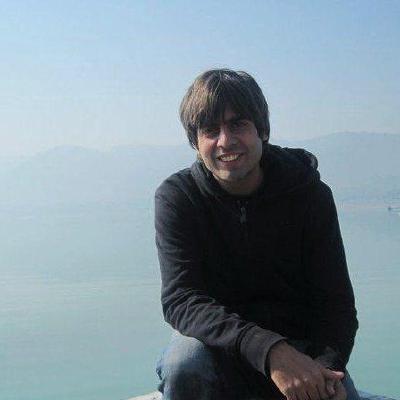
Written by Sahil Batla
Introduction
This article will deal with the issues we face with the current API architecture (mostly REST) and why demand-driven APIs seem a perfect replacement for it. We will also talk in brief, about GraphQL and how it is a feasible solution for implementing demand-driven applications.
Note: This article is inspired from Demand driven Applications with GraphQL by Vinci Rufus at JS Channel 2017.
Why Demand-driven API? What's wrong with REST?
Let's take a simple example of author & articles. If we are given a requirement to develop an API to fetch authors or articles, it will most probably go like this, if we follow REST:
- GET /authors/:authorId
- GET /articles/:articleId
Let's taken an example where we have to show an article snippet on my website's dashboard. We would need its title, description & author name. So we hit the latter end point and it will give a response like:
{
title: 'Demand Driven APIs Using GraphQL',
createdAt: '2017-04-25',
updatedAt: '2017-08-25',
articleId: '96',
authorId: 50,
status: 'published',
description: 'Lorem Ipsum...'
}
There are two problems with this response:
- Extra information: We only needed the title & description but we got everything related to the article and we cannot get rid of this extra payload as this extra information might be getting consumed at some other page i.e. Edit Article Page.
- Missing information: We were expecting author name but instead we got authorId. This is bad and to solve this we would probably be making another network call on the former end point to get the author name. It's an overhead making 2 network calls just to fetch 3 parameters, don't you think? Also, it will just get more complex as we include more resources i.e. comments, images etc.
How Demand-driven Applications Work?
Now that we understand few issues with REST based APIs, we need a smart system which can give me the exact information required instead of giving me partial/extra information.This can be solved if the client demands what it actually needs and server gives it only that piece of information. This can be done using GraphQL.
Let's try to solve our problem using GraphQL. The exact information that our client need can be represented in GraphQL as:
{
article (id: articleId)
{
title,
description,
author {
name
}
}
}
The server can have a single end point with the following schema:
type Article(id: Integer) {
title: String,
description: String,
status: String,
createdAt: Date,
updatedAt: Date,
status: String,
author: Author
}
type Author(id: Integer) {
name: String,
email: String,
photo: Picture,
followers: [User]
}
type Picture(id: Integer) {
imgPath: String,
imgHeight: Integer,
imgWidth: Integer
}
And each field in our schema can have a function to fetch that piece of information. In our case:
function Article(id) {
return Article.find(id);
}
function Article_title(article) {
return article.title;
}
function Article_description(article) {
return article.description;
}
function Article_author(article) {
return article.author;
}
function Author_name(author) {
return author.name;
}
On querying the data, we get i.e.
curl -XGET http://myapp/articles -d "query={
article(id: 1) {
title,
description,
author {
name
}
}
}"
We will get like this:
{
title: 'Demand Driven APIs Using GraphQL',
description: 'Lorem Ipsum...',
author: {
name: 'Sahil Batla'
}
}
This is what we needed, now we can keep the endpoint same and tweak with fields required to display relevant information at any page of our website.
Advantages of Demand-driven APIs
- Single end point for serving any piece of information.
- Less payload of data as no extra information is served.
- Versioning of APIs become simpler as we can control the exact information required.
Disadvantages of Demand-driven APIs
- Latency may increase due to a single end point handling all the querying of data.
- No lazy loading possible as it's a single call which will contain all the data.
Try it Out
If you think GraphQL is promising go ahead and try it out. There is much more to it that you will love to learn. Check out its official documentation. It has been implemented in all the well known languages and you can find it all here.